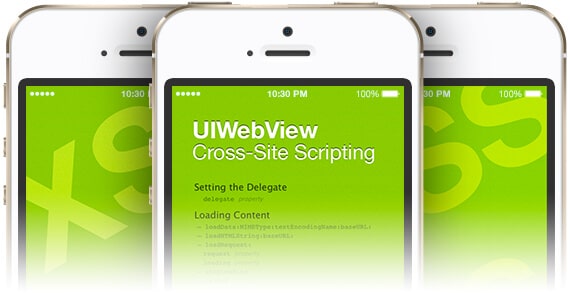
What is iOS UIWebView Cross-Site Scripting (XSS)?
Cross-site scripting occurs when malicious scripts are injected into an otherwise benign or trusted website. Within the mobile security field, cross-site scripting can occur in unlikely places, such as the UIWebView on iOS. For purposes of illustration, we’ll discuss a recent instance of UIWebView cross-site scripting we came across in a test. We’ll also discuss a similar app that does things correctly. Then we’ll cover why problems like this occur and how it’s difficult for developers to foresee these security issues.
UIWebView Example — Vulnerable
The vulnerable native iOS app we tested had an interesting design. User-created content was displayed within a UIWebView. The displaying of user-specified content within UIWebView was not the core of the security issue; the context of how the content was displayed was where security issues were created.
Once we injected JavaScript into the UIWebView we began to explore what cookies and sensitive data were available. Oddly enough, there were no cookies to steal, and no active sessions with the app’s associated web services. This is due to the context differences between a UIWebView and the app’s own cookie jar used to invoke web services. So the problem seemed like a non-exploitable cross-site scripting issue.
That was until we noticed the app was also caching user-generated content locally before building the UIWebView. UIWebView, much like your browser, implements the Document Object Model, or DOM. The security model enforced by the DOM convention prevents abuse-of-functionality attacks that would allow a website to do things like access the client’s local filesystem. When the vulnerable app caches and loads user-generated content into the UIWebView it also unintentionally creates a DOM in the mobile device’s local filesystem. This allows user-generated content access to the filesystem with the same permissions of the iOS app.
To exploit this we used simple JavaScript to read a file’s contents into a variable and then send the variable to a remote server we controlled. We could, for example, read the app’s cookie jar to steal the cookies used to invoke the related web services, or we could grab an entire SQLite database.
UIWebView Example — Secure
An app that has similar functionality but does everything in a secure manner would be Apple’s own mail app. As an experiment we sent HTML files as attachments and then opened the attachments inside the mail app. It’s common for non-mobile mail applications to filter active client-side scripts to prevent attacks; I assume Apple would implement the same type of protection to secure its mobile mail app.
Preventing UIWebView Insecurity
Any app developer that uses UIWebView must consider the context in which the UIWebView is created. If the DOM of the UIWebView allows access to sensitive sessions, or even the local filesystem, the contents of that view must be secured from malicious client-side scripts. If the context of the view contains anything sensitive, then all cross-site scripting vulnerabilities need to be eliminated with the usual techniques of filtering and/or escaping.
Apps that need to display untrusted content inside a UIWebView by design should display that content within a DOM that prevents access to sensitive data. This could be done by hosting the content on a domain that is dedicated to hosting the user-generated content. In addition, make use of the UIWebViewDelegate protocol to implement a whitelist of safe domains to load content from or implement your set of classes to handle the complex problem of whitelisting. A good resource for implementing custom classes would be the Apache Cordova iOS Project (https://github.com/apache/cordova-ios).
The Larger Picture
Apple’s development environments and documentation all fall short in educating developers about the potential dangers of UIWebView. To illustrate this, we’ll take the top Google search results for “UIWebView tutorial” and Apple’s own documentation of UIWebView. Today, the top Google results for that specific search query are:
Link 1: iOS Tutorial – Creating a Web View (UIWebView) Link 2: 31 Days of iOS: Day 14–The UIWebView
Apple’s documentation is located at:
Link 3: iOS Developer Library: UIWebView Class Reference
If we review the likely resources a developer would reference while utilizing UIWebView, we see an alarming lack of security education. The tutorials focus on clicking around in Xcode and writing a couple lines of code; not surprisingly, neither the tutorials nor Xcode attempt to ensure secure code is about to be created. Then reviewing the Apple documentation itself, we can see why these security issues occur. The authoritative documentation for UIWebView doesn’t mention the word “security” once, or even talk about any of the security aspects that govern its behavior.